Take a look at the below code
const int LED = 3; // the output pin for the LED const int BUTTON = 2; // the input pin for the pushbutton int val = 0; // val will be used to store the state // of the input pin int state = 0; // 0 = LED off while 1 = LED on void setup() { pinMode(LED, OUTPUT); // tell Arduino LED is an output pinMode(BUTTON, INPUT); // and BUTTON is an input } void loop() { val = digitalRead(BUTTON); // read input value and store it // check if the input is HIGH (button pressed) // and change the state if (val == HIGH) { state = 1 - state; } if (state == 1) { digitalWrite(LED, HIGH); // turn LED ON } else { digitalWrite(LED, LOW); } }
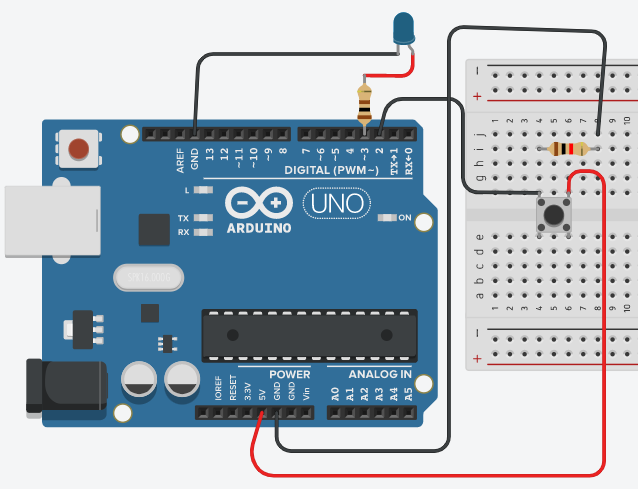
Now test this code by uploading it from Arduino IDE to Arduino. You’ll see that it works… to some extent. You’ll find that the light changes so quickly that you can’t reliably turn it on or off with a single button press.
state is a variable that stores either 0 or 1, to remember whether the LED is on or off. After the button is released, we set it to 0. initialize on
Afterwards, we read the current state of the button, and if it is pressed (val == HIGH), we change the state from 0 to 1, or vice versa.
Later in the program, you can see that we use state to find out whether to turn the LED on or off. As I mentioned, it gives somewhat flaky results.
The results are flaky because of the way we read the button.Arduino is really fast; it executes its own internal instructions at
a rate of 16 million per second—it could well be executing a few million lines of code per second. So this means that while your
finger is pressing the button, Arduino might be reading the button’s position a few thousand times and changing state
accordingly.
The results are flaky because of the way we read the button.Arduino is really fast; it executes its own internal instructions at a rate of 16 million per second—it could well be executing a few million lines of code per second. So this means that while your finger is pressing the button, Arduino might be reading the button’s position a few thousand times and changing state accordingly.
So the results become unpredictable; It can be turned off when you want to turn it on, or vice versa. Since even a broken clock is correct twice a day, the program may show correct behavior each time, but most of the time it will be wrong.
How do you fix this? you need to detect the exact moment when the button is pressed—that is the only moment that you have to change state. The way we like to do it is to store the value of val before we read a new one; this allows you to compare the current position of the button with the previous one and change state only when the button changes from LOW to HIGH.
New and Improved Button Press Code
const int LED = 3; // the output pin for the LED const int BUTTON = 2; // the input pin for the pushbutton int val = 0; // val will be used to store the state // of the input pin int old_val = 0; // this variable stores the previous // value of "val" int state = 0; // 0 = LED off and 1 = LED on void setup() { pinMode(LED, OUTPUT); // tell Arduino LED is an output pinMode(BUTTON, INPUT); // and BUTTON is an input } void loop(){ val = digitalRead(BUTTON); // read input value and store it // yum, fresh // check if there was a transition if ((val == HIGH) && (old_val == LOW)){ state = 1 - state; } old_val = val; // val is now old, let's store it if (state == 1) { digitalWrite(LED, HIGH); // turn LED ON } else { digitalWrite(LED, LOW); } }
You may have noticed that this approach is not entirely perfect, due to another issue with mechanical switches.
As we mentioned earlier, pushbuttons are just two pieces of metal held apart by a spring, which comes into contact when you press switch.
This might seem like the switch should be completely on when you press the button, but in fact what happens is the two pieces of metal bounce o each other, just like a ball bounces on the floor.
Although the bouncing is only for a very small distance and happens for a fraction of a second, it causes the switch to change
between o and on a number of times until the bouncing stops, and Arduino is quick enough to catch this.
When the pushbutton is bouncing, the Arduino sees a very rapid sequence of on and o signals. There are many techniques developed to do debouncing, but in this simple piece of code, it’s usually enough to add a 10- to 50-millisecond delay when the code detects a transition. In other words, you just wait a bit for the bouncing to stop.
Another new and improved code for button presses
const int LED = 3; // the output pin for the LED const int BUTTON = 2; // the input pin for the pushbutton int val = 0; // val will be used to store the state // of the input pin int old_val = 0; // this variable stores the previous // value of "val" int state = 0; // 0 = LED off and 1 = LED on void setup() { pinMode(LED, OUTPUT); // tell Arduino LED is an output pinMode(BUTTON, INPUT); // and BUTTON is an input } void loop(){ val = digitalRead(BUTTON); // read input value and store it // yum, fresh // check if there was a transition if ((val == HIGH) && (old_val == LOW)){ state = 1 - state; delay(50); } old_val = val; // val is now old, let's store it if (state == 1) { digitalWrite(LED, HIGH); // turn LED ON } else { digitalWrite(LED, LOW); } }
If you use ardublockly, save this “xml” code in a notepad. Then change its extension from “.txt” to “.xml”. then open this xml file in ardublockly
<xml xmlns="http://www.w3.org/1999/xhtml"> <block type="arduino_functions" id="NpKcq(ee@?[pG%pm^w(J" x="-9" y="48"> <statement name="SETUP_FUNC"> <block type="variables_set" id="8HQG(by(v-K^;`0BM|H3"> <field name="VAR">val</field> <value name="VALUE"> <block type="variables_set_type" id="r@q+T!`i]`QBt~-F:3N2"> <field name="VARIABLE_SETTYPE_TYPE">BOOLEAN</field> <value name="VARIABLE_SETTYPE_INPUT"> <block type="math_number" id="{5P8rh9rVcyB5Soy*~RN"> <field name="NUM">0</field> </block> </value> </block> </value> <next> <block type="variables_set" id="|y0u|;~|JWVe!z:Gd}5V"> <field name="VAR">old_val</field> <value name="VALUE"> <block type="variables_set_type" id="90;[Xbg!HgXiVWa[QCEN"> <field name="VARIABLE_SETTYPE_TYPE">BOOLEAN</field> <value name="VARIABLE_SETTYPE_INPUT"> <block type="math_number" id="eBpoRm,x)@wYMek}vm#;"> <field name="NUM">0</field> </block> </value> </block> </value> <next> <block type="variables_set" id="e%NOlE[WW)^lB^RfQ9n_"> <field name="VAR">state</field> <value name="VALUE"> <block type="variables_set_type" id=".@%PrP{J[LlBBunK1jf%"> <field name="VARIABLE_SETTYPE_TYPE">BOOLEAN</field> <value name="VARIABLE_SETTYPE_INPUT"> <block type="math_number" id="{gj,R~Kcu@+iJG/#{T.d"> <field name="NUM">0</field> </block> </value> </block> </value> </block> </next> </block> </next> </block> </statement> <statement name="LOOP_FUNC"> <block type="variables_set" id="6h3B2ut@|D*{hoTO7qqb"> <field name="VAR">val</field> <value name="VALUE"> <block type="io_digitalread" id="CfeasS5X9;5Uf/huF0xs"> <field name="PIN">2</field> </block> </value> <next> <block type="controls_if" id="x%S(d{rqiS^H+/L-NewY"> <value name="IF0"> <block type="logic_operation" id="Ddp.|~{Db#|5}0O=rO{w"> <field name="OP">AND</field> <value name="A"> <block type="logic_compare" id="q[Y+Z(MdcDjjkHr?65W;"> <field name="OP">EQ</field> <value name="A"> <block type="variables_get" id="[_WeAM*J:~8fc:ps,[%d"> <field name="VAR">val</field> </block> </value> <value name="B"> <block type="logic_boolean" id="09OX%5kv/qI80CZPk:{K"> <field name="BOOL">TRUE</field> </block> </value> </block> </value> <value name="B"> <block type="logic_compare" id="[email protected]#s{!Pbs1^"> <field name="OP">EQ</field> <value name="A"> <block type="variables_get" id="Lu[P4:EJs{[P(x-yLp[7"> <field name="VAR">old_val</field> </block> </value> <value name="B"> <block type="logic_boolean" id="P-a*p]3`-u]ubtN%3I@/"> <field name="BOOL">FALSE</field> </block> </value> </block> </value> </block> </value> <statement name="DO0"> <block type="variables_set" id="5w73ClA^qD1L}Tr%d[+~"> <field name="VAR">state</field> <value name="VALUE"> <block type="logic_negate" id="?ih{^1SRS[=P@Ih/O(0I"> <value name="BOOL"> <block type="variables_get" id="z/9)%!a!.jZ*yIKtx@iu"> <field name="VAR">state</field> </block> </value> </block> </value> <next> <block type="time_delay" id="!w+78vdu{km!QnP8YQ|N"> <value name="DELAY_TIME_MILI"> <block type="math_number" id="%]vScUOijO=:N?}:lP3n"> <field name="NUM">50</field> </block> </value> </block> </next> </block> </statement> <next> <block type="variables_set" id="p-:vyo?3nubW.?/h?kC;"> <field name="VAR">old_val</field> <value name="VALUE"> <block type="variables_get" id="YBu2@yp4HK}r:fy[_-_6"> <field name="VAR">val</field> </block> </value> <next> <block type="controls_if" id="26_1x+1@*ry9b4~LEB3u"> <mutation else="1"></mutation> <value name="IF0"> <block type="logic_compare" id="BD/pnpN+RK{d@hA0]hRV"> <field name="OP">EQ</field> <value name="A"> <block type="variables_get" id="`Yi4u`wT#p98Pf26Ke2A"> <field name="VAR">state</field> </block> </value> <value name="B"> <block type="logic_boolean" id="+`d3@~E.)R9Xdeeu{#1c"> <field name="BOOL">TRUE</field> </block> </value> </block> </value> <statement name="DO0"> <block type="io_digitalwrite" id="qp5?2WDcsR;M*NF{(f(M"> <field name="PIN">3</field> <value name="STATE"> <block type="io_highlow" id="x.GO+hMJf34U-~[|apDp"> <field name="STATE">HIGH</field> </block> </value> </block> </statement> <statement name="ELSE"> <block type="io_digitalwrite" id="(zwu+IuWEm`Ayyx[Hp!T"> <field name="PIN">3</field> <value name="STATE"> <block type="io_highlow" id="]|u.;Iy7..=?Wz[oq^Nr"> <field name="STATE">LOW</field> </block> </value> </block> </statement> </block> </next> </block> </next> </block> </next> </block> </statement> </block> </xml>