Code to change the brightness as you hold the pushbutton
const int LED = 3; // the pin for the LED const int BUTTON = 2; // input pin of the pushbutton int val = 0; // stores the state of the input pin int old_val = 0; // stores the previous value of "val" int state = 0; // 0 = LED off while 1 = LED on int brightness = 128; // Stores the brightness value unsigned long startTime = 0; // when did we begin pressing? void setup() { pinMode(LED, OUTPUT); // tell Arduino LED is an output pinMode(BUTTON, INPUT); // and BUTTON is an input } void loop() { val = digitalRead(BUTTON); // read input value and store it // yum, fresh // check if there was a transition if ((val == HIGH) && (old_val == LOW)) { state = 1 - state; // change the state from off to on // or vice-versa startTime = millis(); // millis() is the Arduino clock // it returns how many milliseconds // have passed since the board has // been reset. // (this line remembers when the button // was last pressed) delay(10); } // check whether the button is being held down if ((val == HIGH) && (old_val == HIGH)) { // If the button is held for more than 500 ms. if (state == 1 && (millis() - startTime) > 500) { brightness++; // increment brightness by 1 delay(10); // delay to avoid brightness going // up too fast if (brightness > 255) { // 255 is the max brightness brightness = 0; // if we go over 255 // let's go back to 0 } } } old_val = val; // val is now old, let's store it if (state == 1) { analogWrite(LED, brightness); // turn LED ON at the // current brightness level } else { analogWrite(LED, 0); // turn LED OFF } }
Try it now.
If you press the button and release it immediately, you switch the lamp on or o. If you hold the button down, the brightness changes; just let go when you have reached the desired brightness.
Before thinking about the circuit, try to spend a little time trying to understand the program. Perhaps the most confusing line is this:
if (state == 1 && (millis() - startTime) > 500) {
This checks to see if the button is held down for more than 500ms by using a built-in function called millis(), which is just a running count of the number of milliseconds since your sketch started running. By keeping track of when the button was pressed (in the variable startTime), we can compare the current time to the start time to see how much time has passed.
Of course, this only makes sense if the button is currently pressed, which is why at the beginning of the line we check to see if ‘state’ is set to a value of 1.
Use a Light Sensor Instead of the Pushbutton
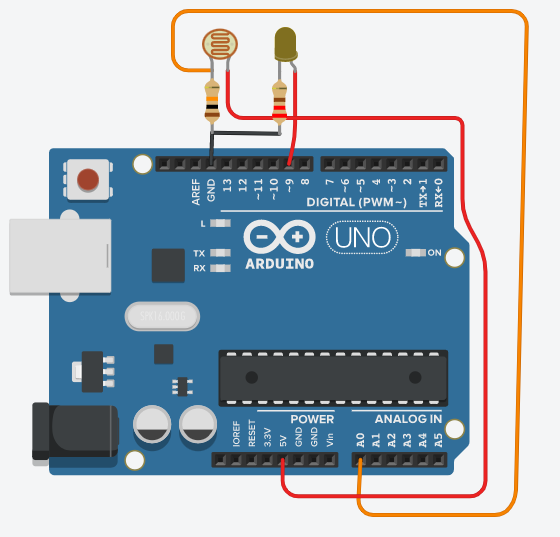
int ldr_val = 0; // variable used to store the value coming from the sensor void setup() { pinMode(9, OUTPUT); // Note: Analogue pins are automatically set as inputs } void loop() { ldr_val = analogRead(A0); // read the value from the sensor analogWrite(9, (ldr_val / 4)); delay(10); // Wait for 10 millisecond(s) }
Once it’s running, cover and uncover the LDR and see what happens to the LED brightness.
We specify the brightness by dividing ldr_val by 4, because analogRead() returns a number up to 1023,
and analogWrite() accepts a maximum of 255.